You can view this finished project live at passgen.site.
Getting the idea
I wanted to create a project that would be fun to work on and put my javascript skills to the test. Creating a password generator is a common beginner’s programming project, but this challenged me to create a password generator that was different and better than all others.
I was inspired by this XKCD comic:
From the comic, I got that sentence-based passwords are easier to remember and are more secure. I came across this password generator on Github that does just that. It generated these two sentence-based passwords:
- Wherever-office-pocket-committee
- Pincushion-science-without-civilization
However, most password generators I came across generated a random string of characters. Some consisted of only letters and numbers, for example random.org generated these:
- MB3VsXU3
- YLSMYYDN
And some websites additionally used symbols, non-alphabetic, non-numeric characters. For example, passwordsgenerator.net generated these:
- -n&7y&9$]85K{t9c
- ~Zau;U/]Q5\&L4H6
I also came across a few pronounceable password generators. These don't use actual words, but are constructed of pronouncable syllables. For example, lastpass.com generated these:
- QuInDurafToN
- quiShAnisEpo
Now, if I align all these passwords, you might see a trend:
- Correct-horse-battery-staple
- Wherever-office-pocket-committee
- Pincushion-science-without-civilization
- QuInDurafToN
- quiShAnisEpo
- MB3VsXU3
- YLSMYYDN
- -n&7y&9$]85K{t9c
- ~Zau;U/]Q5\&L4H6
I would describe the trend as a transition from a complete sentence to a random combination of characters. This is how I designed my password generator to function. I created a slider (HTML input range) that deteriorates sentence-based passwords, allowing the user to choose any "recipe" for the password with a single UI control.
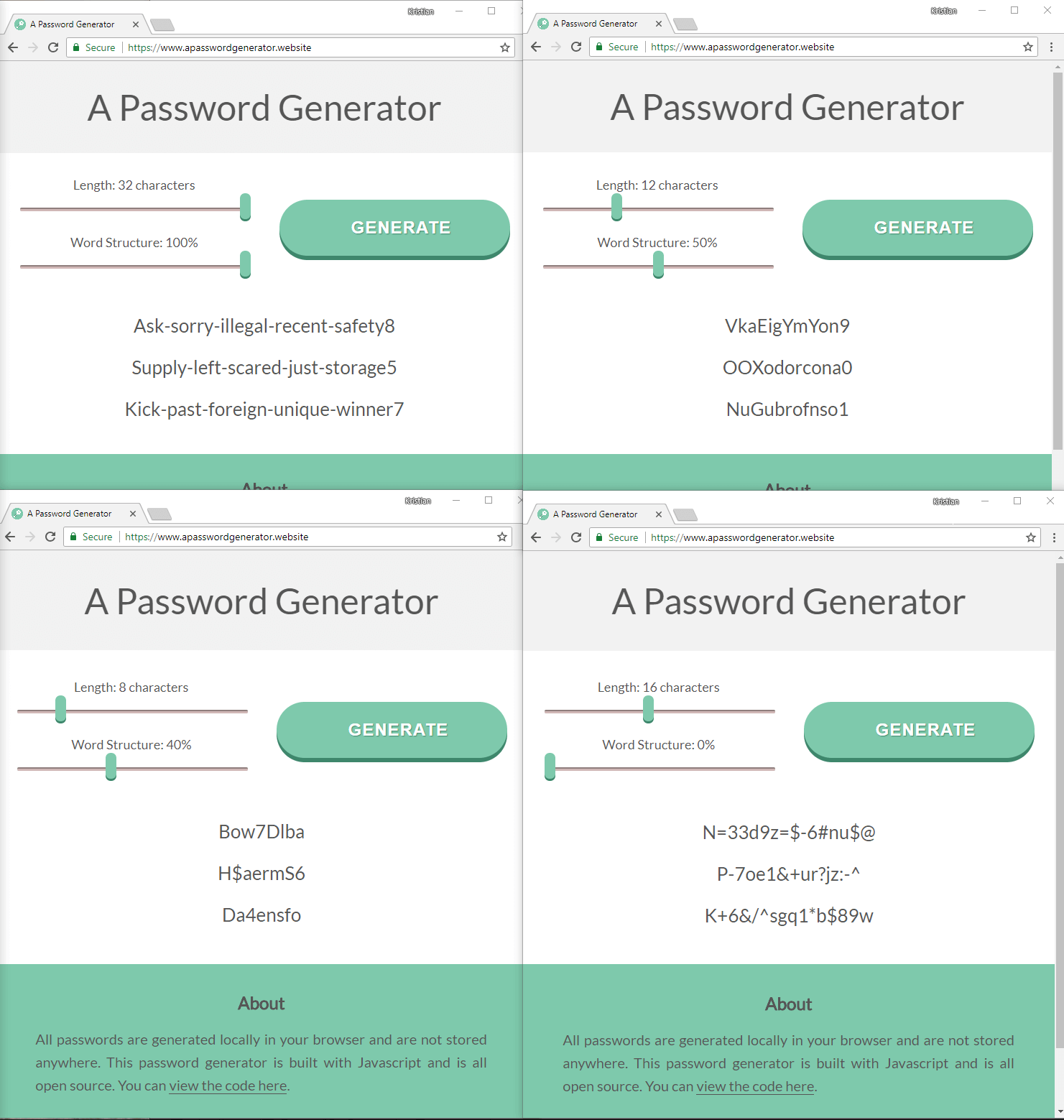
My website is capable of reproducing all of the different "recipes" of passwords: Sentence-based passwords, pronouncable passwords, alpha-numeric passwords, and random character passwords.
How the script functions
The script functions by creating a sentence in the format "verb-adjective-adjective-adjective-noun-number", and is broken down into 11 "steps"; in each of which some characters are replaced. Each character is replaced twice from steps 0-10, chosen at random.
For example, in a 32-character password, each of the 32 character positions will hold 3 different characters depending on which step you are on (which is determined by the user-controlled slider). Here is a brief description of the passwords it generates:
- Step 10: Full sentence.
- Steps 7-9: Pronouncable sentence.
- Steps 4-6: Random letters and numbers.
- Steps 0-3: Random symbols, letters, and numbers.
Here is an example of a generated password:
- Step 10: Accuse-visual-dirty-huge-dinner0
- Step 9: Accosa-visoal-dirty-huga-dinnar0
- Step 8: Ackoza-visoal-derty-huga-denmar0
- Step 7: Askoza-vivoil-dergy-dola-denmar0
- Step 6: Yskoza5nivoil8deggubdolaydepmar0
- Step 5: Yskoza5nEvoiA8EeggubdolayKepmaL6
- Step 4: YskozafjEvo9j83egwubdolayxepmaL6
- Step 3: YskozafjElo9j83etwubdola2xe84aLw
- Step 2: Vs0od$fj8lo9j83etwubdola2xo84aLw
- Step 1: V&0{d$fj8li9j83etwubd@na2xo84/{w
- Step 0: V&0{d$fj8li9j-3,tw;%e@nk2xo84/{w
This is the functionality of the word structure slider. The other slider determines the length, which has some requirements:
- Pronounceable passwords should be kept pronounceable.
- At least one uppercase letter.
- At least one number.
To keep pronounceable passwords pronounceable, the script replaces the cut-off word with a set of numbers:
- 32 chars: Accuse-visual-dirty-huge-dinner0
- 31 chars: Accuse-visual-dirty-huge-012234
- 30 chars: Accuse-visual-dirty-huge-01223
- 29 chars: Accuse-visual-dirty-huge-0122
- 28 chars: Accuse-visual-dirty-huge-012
- 27 chars: Accuse-visual-dirty-huge-01
- 26 chars: Accuse-visual-dirty-huge-0
- 25 chars: Accuse-visual-dirty-huge3
- 24 chars: Accuse-visual-dirty-8293
- 23 chars: Accuse-visual-dirty-829
- 22 chars: Accuse-visual-dirty-82
- 21 chars: Accuse-visual-dirty-8
- 20 chars: Accuse-visual-dirty7
- 19 chars: Accuse-visual-01417
- 18 chars: Accuse-visual-0141
- 17 chars: Accuse-visual-014
- 16 chars: Accuse-visual-01
- 15 chars: Accuse-visual-0
- 14 chars: Accuse-visual1
- 13 chars: Accuse-415241
- 12 chars: Accuse-41524
- 11 chars: Accuse-4152
- 10 chars: Accuse-415
- 09 chars: Accuse-41
- 08 chars: Accuse-4
- 07 chars: Accuse3
- 06 chars: Accus0
- 05 chars: Accu0
- 04 chars: Acc0
- 03 chars: Ac0
User Experience (UX)
I decided to change the position of elements between mobile and desktop devices for the best user experience across platforms.
On a laptop or desktop, the placement of elements is determined by the logical flow of events:
- 1. Adjust the sliders.
- 2. Click generate.
- 3. Review or copy the password.
The three elements are positioned following the traditional direction of reading: left-to-right and top-to-bottom.
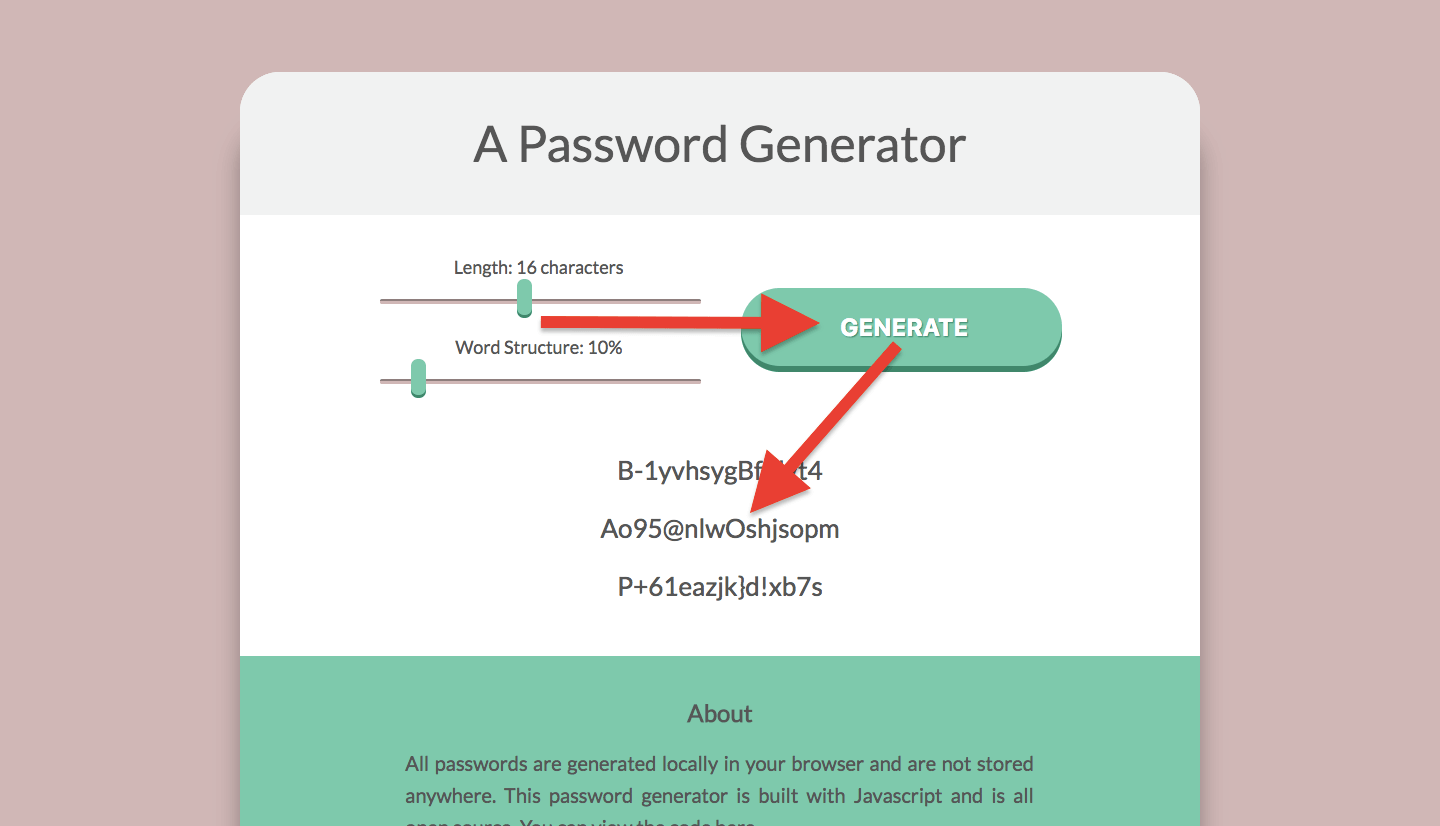
However, accessbility is a higher priority on mobile devices. Here is an image of thumb-zone mapping that shows the accessibility of content on mobile devices:
If you are interested in learning more about mobile UX, this image is from The Thumb Zone: Designing For Mobile Users.
In order to provide the best user experience, high-frequency-interaction elements should be the most accessible, while low-frequency-interaction elements should be the least accessible.
On mobile, the placement of elements is determined by the frequency of the element being used (anchored to the bottom):
- 1. Generate button
- 2. Slider controls
- 3. Passwords
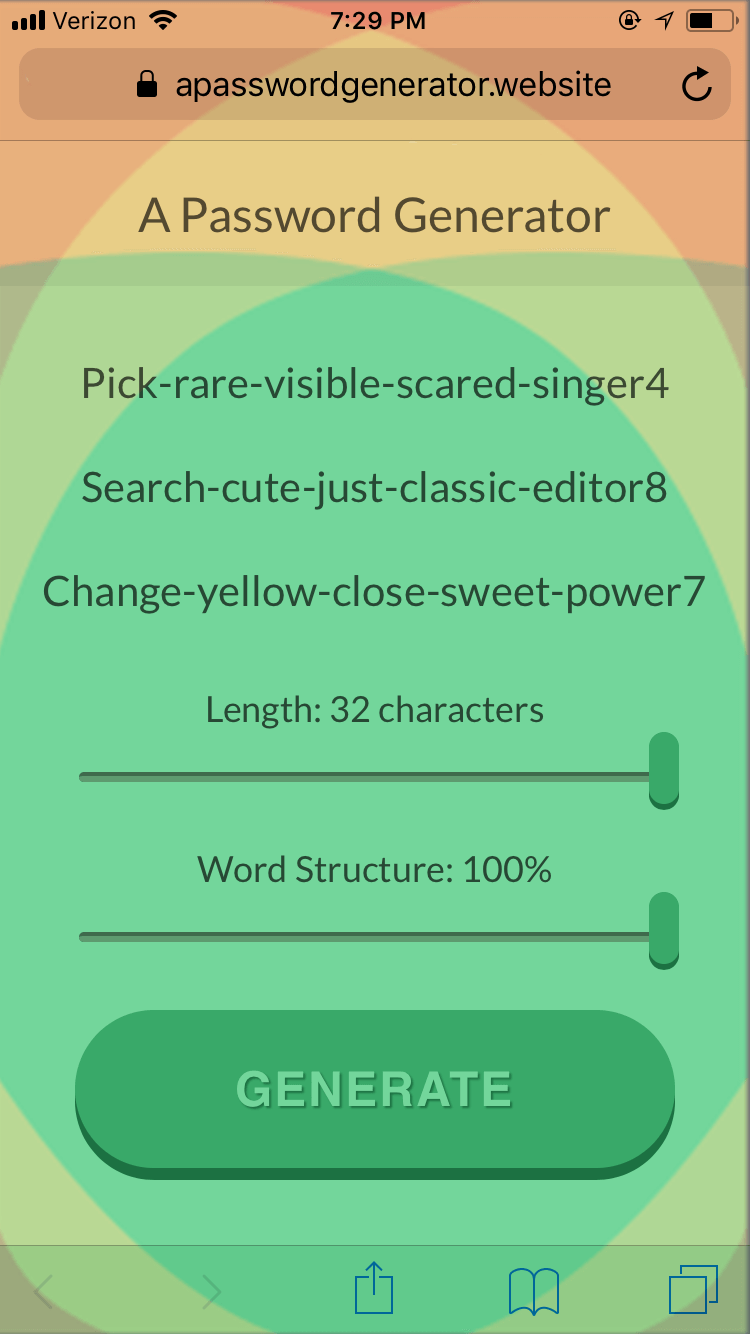
In this image, all elements are within the green-natural region, so the user doesn't have to scroll or extend their thumb to use the app.
Conclusion
My password generator has all of the functionality of other password generators but provides a better user experience.
It is the first password generator to use 2 sliders as the only UI controls, and the first to create both sentence-based passwords and random-character-based passwords.
Working on this project got me thinking outside the box as I reimagined the way users interact with the app. I had fun while gaining valuable experience with javascript and UX.
You can view all the code for this project on Github: github.com/KristianWindsor/passgen.site
Thanks for reading!